How to Compile a Program into Different Projects in C#
How to Compile a Program into Different Projects in C#
In the world of software development, C# Compiler plays a crucial role in transforming human-readable code into machine-executable instructions. As projects grow in complexity, developers often find themselves needing to compile programs into different projects within a single solution. This comprehensive guide will walk you through the process of compiling a program into different projects in C#, providing valuable insights for both beginners and experienced programmers. Whether you’re preparing for c# interview questions for freshers or looking to enhance your skills, understanding this concept is essential for efficient software development.
Understanding the Basics of C# Project Compilation
Before diving into the intricacies of compiling programs into different projects, it’s crucial to grasp the fundamentals of C# compilation. The C# compiler takes your source code and transforms it into intermediate language (IL) code, which is then executed by the Common Language Runtime (CLR). This process ensures that your C# programs can run on any platform that supports the .NET framework.
The Role of the C# Compiler
The C# Compiler is a powerful tool that performs several important tasks:
- Syntax checking: It verifies that your code adheres to C# language rules.
- Type checking: It ensures that variables and methods are used correctly according to their defined types.
- Code optimization: It improves the performance of your code by making it more efficient.
- IL code generation: It produces the intermediate language code that can be executed by the CLR.
Understanding these basic functions will help you appreciate the compiler’s role in multi-project solutions.
Creating a Multi-Project Solution in C#
When working on large-scale applications, it’s common to divide your codebase into multiple projects within a single solution. This approach offers several benefits, including:
- Better organization of code
- Improved maintainability
- Enhanced reusability of components
- Easier collaboration among team members
Steps to Create a Multi-Project Solution
- Open Visual Studio and create a new solution.
- Add multiple projects to the solution (e.g., a main application project, library projects, and test projects).
- Set up project dependencies and references as needed.
Compiling Programs into Different Projects
Now that we have a multi-project solution set up, let’s explore how to compile programs into different projects effectively.
Understanding Project Dependencies
In a multi-project solution, projects often depend on each other. For example, your main application project might rely on functionality provided by a library project. To ensure successful compilation, you need to:
- Add project references: Right-click on the project that needs to use another project’s code and select “Add Reference.”
- Manage NuGet packages: Ensure that all required packages are installed and up-to-date across your projects.
Configuring Build Orders
The build order of your projects is crucial when compiling a program into different projects. To set the correct build order:
- Right-click on your solution in the Solution Explorer.
- Select “Project Build Order.”
- Arrange the projects so that dependencies are built before the projects that rely on them.
Using Conditional Compilation
Conditional compilation allows you to include or exclude portions of code based on certain conditions. This technique is particularly useful when compiling programs into different projects. Here’s how you can use it:
csharp
Copy
#if DEBUG
Console.WriteLine(“This code will only be compiled in debug mode”);
#endif
#if RELEASE
Console.WriteLine(“This code will only be compiled in release mode”);
#endif
By using conditional compilation, you can tailor your code to specific project configurations or target platforms.
Advanced Techniques for Multi-Project Compilation
As you become more proficient in compiling programs into different projects, you’ll want to explore some advanced techniques to streamline your development process.
Utilizing Shared Projects
Shared projects allow you to share code between multiple projects without creating a separate assembly. To use shared projects:
- Add a new Shared Project to your solution.
- Move common code into the shared project.
- Add a reference to the shared project in the projects that need to use the shared code.
This approach can significantly reduce code duplication and improve maintainability.
Leveraging Project Templates
Creating custom project templates can save you time when setting up new projects within your solution. To create a project template:
- Develop a project with the desired structure and initial code.
- Export the project as a template (Project > Export Template).
- Use the template when adding new projects to your solution.
Implementing Continuous Integration (CI)
Continuous Integration can greatly enhance your multi-project compilation process. By automatically building and testing your projects whenever changes are pushed to your version control system, you can catch and fix issues early in the development cycle.
Popular CI tools for C# projects include:
- Azure DevOps
- Jenkins
- TeamCity
- GitHub Actions
Setting up CI for your multi-project solution ensures that all projects compile correctly and pass tests before merging changes into the main codebase.
Best Practices for Compiling Programs into Different Projects
To make the most of compiling programs into different projects, consider the following best practices:
- Keep project responsibilities clear: Each project should have a well-defined purpose and scope.
- Minimize circular dependencies: Avoid creating circular references between projects, as they can lead to compilation issues.
- Use consistent naming conventions: Adopt a clear naming strategy for projects, namespaces, and classes across your solution.
- Optimize build configurations: Tailor your build configurations to different environments (e.g., debug, release, staging).
- Regularly update dependencies: Keep your project references and NuGet packages up-to-date to avoid compatibility issues.
Troubleshooting Common Compilation Issues
Even with careful planning, you may encounter compilation issues when working with multi-project solutions. Here are some common problems and their solutions:
Missing References
If you’re getting errors about missing types or namespaces, double-check your project references and NuGet packages. Ensure that all required dependencies are properly linked and up-to-date.
Circular Dependencies
Circular dependencies occur when two or more projects reference each other, creating a loop. To resolve this:
- Identify the circular dependency.
- Refactor your code to break the cycle, possibly by introducing an interface or a third project.
Inconsistent Versions
When different projects in your solution use different versions of the same library, it can lead to compilation errors. To fix this:
- Standardize library versions across your projects.
- Use NuGet package management to ensure consistency.
Future Trends in C# Compilation
As C# and .NET continue to evolve, we can expect to see advancements in compilation techniques and tools. Some emerging trends include:
- Improved cross-platform compilation with .NET Core and .NET 5+.
- Enhanced support for cloud-native development and containerization.
- Integration of AI-assisted coding and compilation optimization.
Staying informed about these trends will help you adapt your multi-project compilation strategies to future developments in the C# ecosystem.
Conclusion
Compiling a program into different projects in C# is a valuable skill that can significantly improve your software development workflow. By understanding the compilation process, leveraging multi-project solutions, and implementing best practices, you can create more maintainable, efficient, and scalable applications. Remember that mastering this aspect of C# development is an ongoing process, and staying up-to-date with the latest tools and techniques is crucial for success in the ever-evolving world of software engineering.
Whether you’re a beginner tackling c# interview questions for freshers or an experienced developer working on complex enterprise solutions, the ability to effectively compile programs into different projects will serve you well throughout your career. Keep experimenting, learning, and refining your skills to become a true expert in C# multi-project compilation.
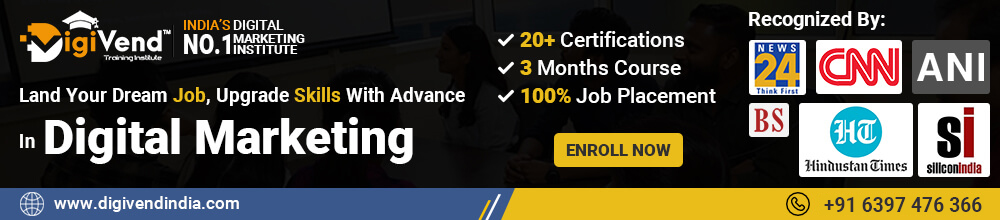