Coding Names: A In-depth Instructional Booklet
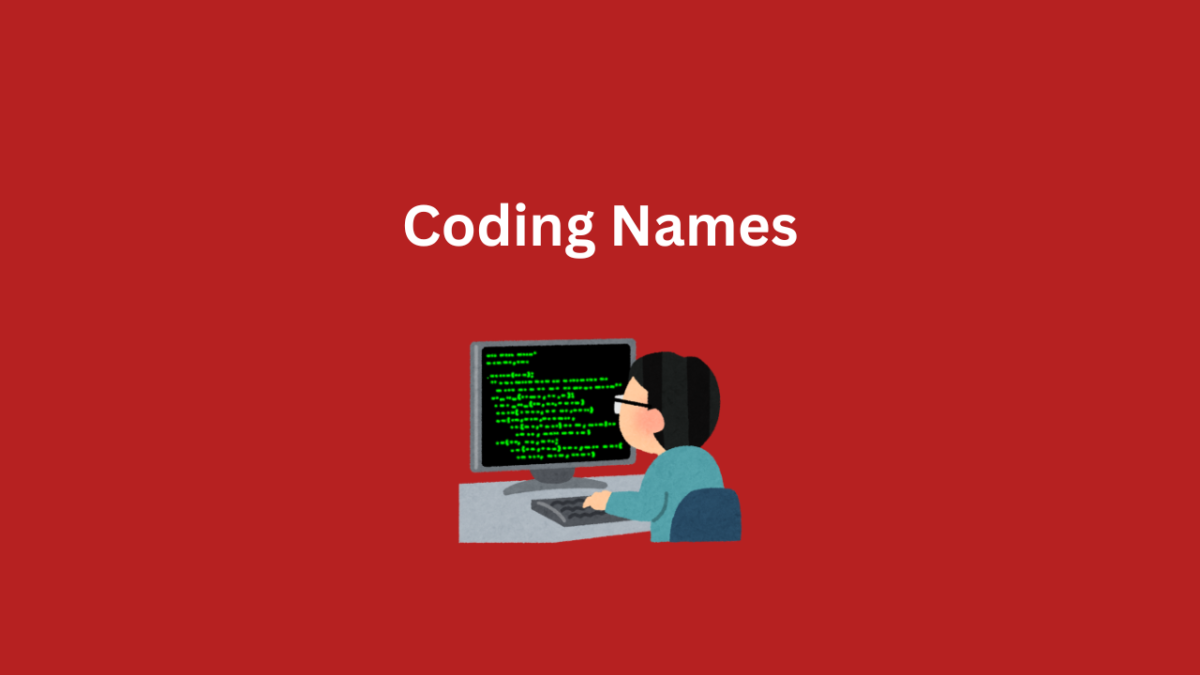
In this article, we will look deeper into the importance of coding names, highlighting their significant role in programming clarity, maintenance, and overall success. We will discuss why coding names are crucial, share best practices, identify common pitfalls, and provide further insights on this topic.
Why Coding Names Matter
Clarity and Readability
Consider envisioning the experience of reading a book in which every character is denoted by the letters “A”, “B”, and “C”. Confusing, right? The same goes for code. Clear and descriptive names make your code more readable and understandable. When you or someone else looks at the code later, the purpose and function of each part should be immediately apparent.
Maintenance and Scalability
Good naming conventions make maintaining and scaling your codebase much easier. As your project grows, having a consistent and logical naming system helps you manage complexity. It allows you to add new features, fix bugs, and refactor code without getting lost in a sea of vague names.
Team Collaboration
Consistent naming conventions are crucial when collaborating in a team as they promote clarity and understanding. By adhering to these conventions, potential misunderstandings are minimized, and the development process becomes more efficient. This allows teammates to easily comprehend the purpose of various variables, functions, and classes, resulting in improved collaboration and accelerated development cycles.
Basic Principles of Naming Conventions
Descriptive and Specific
Names should clearly describe what the variable, function, or class represents. Avoid generic names like “data” or “temp”. Instead, use names that convey the specific purpose, like “userData” or “tempFile”.
Consistency
It is crucial to maintain a consistent naming style across your codebase. Whether you opt for camelCase, snake_case, or any other convention, adhering to consistency greatly contributes to the readability and professionalism of your code.
Avoiding Ambiguity
Names should be unambiguous and straightforward. It is advisable to refrain from using names that have multiple interpretations. For example, the term “count” is more precise than “num” as it explicitly indicates what is being counted.
Types of Coding Names
Variable Names
Variables store data that can change over time. Their names should indicate the type of data and its purpose. For example, “userAge” or “isAuthenticated”.
Function Names
Functions perform actions, so their names should include verbs. Good examples are “calculateTotal” or “fetchUserData”.
Class Names
Classes often represent objects or entities, so they should be named using nouns. Examples include “User” or “Invoice”.
Constant Names
Constants hold values that do not change. Their names are typically in all caps, like “MAX_USERS” or “PI”.
Best Practices for Variable Names
Using Descriptive Names
Choose names that describe what the variable holds. For example, instead of “x” or “y”, use “width” or “height”.
Avoiding Single Letters
Single-letter names are only useful in specific contexts, such as loop indices. For most variables, longer, descriptive names are better.
Using CamelCase or snake_case
Select a naming style and stick with it. In numerous programming languages, it is common to use CamelCase (e.g., “userAge”), whereas snake_case (e.g., “user_age”) is the preferred naming convention in languages like Python.
Effective Function Names
Action-Oriented Names
Functions should have names that reflect their action. For instance, “sendEmail” or “updateProfile”.
Using Verbs and Nouns
Combining verbs with nouns makes function names more descriptive. Examples include “createUser” or “deleteRecord”.
Keeping Names Short but Descriptive
While names ought to possess descriptive qualities, they should also maintain conciseness. Strive for a harmonious equilibrium that imparts sufficient information without becoming excessively wordy.
Naming Classes and Objects
Singular Nouns for Classes
Class names should typically be singular nouns, such as “Product” or “Order”. This convention reflects that each instance of the class represents a single entity.
Capitalizing Class Names
Most programming languages recommend capitalizing the first letter of class names, following the PascalCase convention, like “Customer” or “InvoiceItem”.
Using Prefixes and Suffixes
Prefixes and suffixes can add clarity. For example, “Abstract” as a prefix for abstract classes or “Impl” for implementation classes, like “AbstractShape” or “UserImpl”.
Constants and Enumerations
All Caps for Constants
Constants should be easily distinguishable from variables. Using all caps, such as “DEFAULT_TIMEOUT” or “MAX_CONNECTIONS”, makes them stand out.
Using Underscores for Separation
For readability, use underscores to separate words in constant names, like “TOTAL_COUNT” or “MIN_VALUE”.
Clear and Descriptive Names
Even though constants are often simple values, their names should still be clear and descriptive. “SECONDS_IN_A_MINUTE” is more informative than “SIAM”.
Common Mistakes in Coding Names
Overly Abbreviated Names
Avoid excessive abbreviations that obscure meaning. “cnt” might save a few keystrokes but “count” is much clearer.
Inconsistent Naming Conventions
Switching between naming conventions can cause confusion. Consistency is key to maintaining readable and manageable code.
Using Reserved Words
Using language-specific reserved words as names can lead to errors and confusion. Avoid names like “class”, “int”, or “function”.
Tools and Resources for Naming Conventions
Linters and Code Analyzers
Tools like ESLint for JavaScript or Pylint for Python can enforce naming conventions and catch inconsistencies early.
Documentation and Style Guides
Refer to official documentation and community style guides for best practices. They provide valuable insights into naming conventions specific to each language.
Code Reviews and Peer Feedback
Regular code reviews and peer feedback can help identify and correct poor naming practices, fostering a culture of continuous improvement.
Case Study: Naming Conventions in Popular Frameworks
JavaScript (React, Angular)
In React, component names are usually capitalized (PascalCase), like “UserProfile”. Angular follows similar conventions, with services typically suffixed by “Service”, such as “UserService”.
Python (Django, Flask)
Django and Flask emphasize readability with snake_case for functions and variables, while class names use PascalCase, like “UserSerializer” or “UserModel”.
Python code can be compiled by online compilers like Python online compiler.
Java (Spring, Hibernate)
Java’s Spring framework uses camelCase for variables and methods, while classes are in PascalCase. Hibernate follows similar conventions, with entities typically named using nouns.
Adapting Naming Conventions to Different Languages
Dynamic vs. Static Typing
Languages like JavaScript (dynamic typing) often have different conventions compared to Java (static typing). Understand the idioms of each language you work with.
Language-Specific Conventions
Each programming language has its own conventions. For instance, Python prefers snake_case, while Java favors camelCase.
Community Standards
Adhering to community standards ensures your code aligns with widely accepted practices, making it easier for others to read and contribute.
The Future of Naming Conventions
AI and Automated Naming
AI tools are emerging that can suggest or even automatically generate consistent and meaningful names, potentially revolutionizing coding practices.
Evolution with New Programming Paradigms
As programming paradigms progress, naming conventions will also evolve. Staying updated with these advancements guarantees that your code stays contemporary and easily understandable.
Conclusion
Wen it comes to coding names, good naming conventions are the unsung heroes of clean, maintainable, and collaborative code. By following best practices and avoiding common pitfalls, you can significantly improve the quality and readability of your code. Remember, the goal is to make your code self-explanatory and a joy to work with.
FAQs
Why are coding names important?
Coding names are crucial for clarity, readability, and maintainability of the code. They help developers understand the code quickly and make collaboration easier.
What are some common naming conventions?
Common naming conventions include using camelCase or snake_case for variables, PascalCase for class names, and all caps for constants.
How can I improve my naming conventions?
To improve your naming conventions, follow best practices like being descriptive, consistent, and avoiding ambiguity. Regularly review your code and seek feedback from peers.
What tools can help with naming conventions?
Tools like linters (e.g., ESLint for JavaScript, Pylint for Python) and code analyzers can enforce naming conventions and highlight inconsistencies.
Are there differences in naming conventions between programming languages?
Various programming languages adhere to specific conventions. For instance, Python utilizes snake_case, whereas Java leans towards camelCase. It is crucial to adhere to the conventions of the language being utilized.
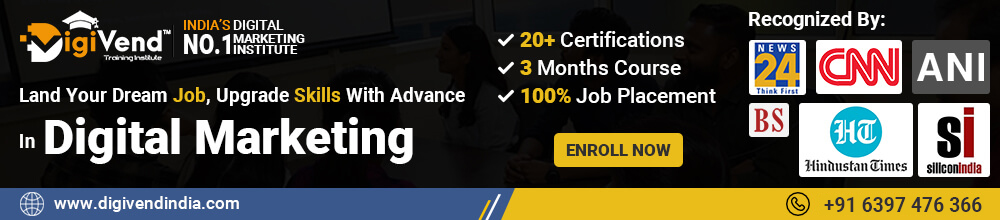