Building a Node.js CLI Tool for Automating Tasks
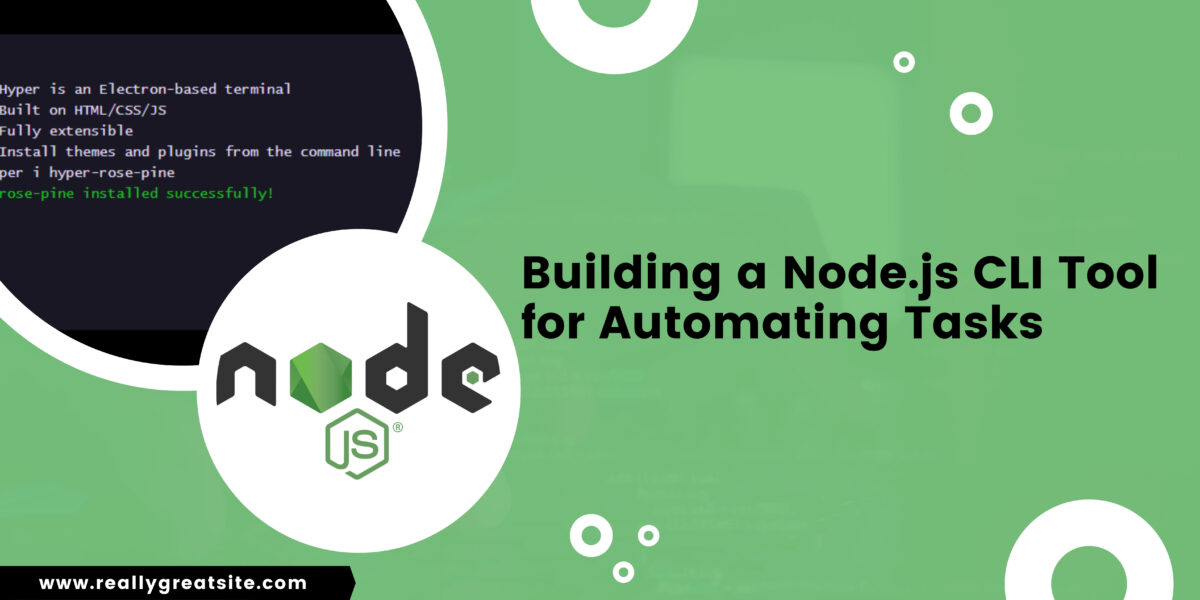
Have you ever found yourself tediously repeating the same tasks on your computer? Maybe it’s renaming a bunch of files, copying specific folders, or running routine system checks. If so, you’ve just encountered the perfect use case for a Command-Line Interface (CLI) tool.
If you’ve ever found yourself tediously repeating the same tasks on your computer—whether it’s renaming a bunch of files, copying specific folders, or running routine system checks—you’ve just encountered the perfect use case for a Command-Line Interface (CLI) tool.
To get started with leveraging CLI tools efficiently, it’s essential to have Node.js and npm installed on your system. Here’s how to install Node.js and npm:
-
Download Node.js: Visit the official Node.js website at nodejs.
- Click on the “Downloads” button to access the downloads page.
- Choose the appropriate installer for your operating system (Windows, macOS, or Linux).
- Download and run the installer, following the on-screen instructions.
-
Verify Installation: After installing Node.js, you can verify that it was installed correctly by opening a terminal or command prompt and typing the following commands:
node -v npm -v
These commands will display the installed versions of Node.js and npm, respectively.
Once you have Node.js and npm installed, you can easily install and manage CLI tools like file renaming utilities, folder manipulation scripts, or system maintenance scripts using npm packages. This enables you to automate repetitive tasks and streamline your workflow efficiently.
What are CLI Tools and Why Use Them?
CLI tools, also known as command-line interfaces, are programs you interact with directly through text commands in your terminal. They offer a powerful way to automate repetitive tasks, saving you valuable time and effort. Imagine a single command that renames all your vacation photos or compresses a folder full of documents – that’s the magic of CLIs!
Building with Node.js: JavaScript Powerhouse for CLI Tools
So, why choose Node.js for building your own CLI tool? Here’s the beauty: Node.js leverages JavaScript, a language many developers are already familiar with. This familiarity translates to a smoother development experience. Additionally, Node.js applications are inherently cross-platform, meaning they run seamlessly on Windows, macOS, and Linux systems.
Ready to Get Started? Let’s Build a Basic CLI Tool!
This guide will walk you through creating a simple Node.js CLI tool. We’ll focus on core functionality, but feel free to explore further customization later.
Prerequisites:
- Node.js and NPM installed: Having Node.js and its package manager, NPM (Node Package Manager), is essential. If you don’t have them yet, head over to https://nodejs.org/en to download and install the latest version.
Setting Up Your Project:
- Create a project directory: Open your terminal and navigate to your desired location using the
cd
command. Then, create a new directory for your project:Bashmkdir my-cli-tool cd my-cli-tool
Use code with caution content_copy - Initialize a Node.js project: Within your project directory, use NPM to initialize a basic Node.js project structure:Bash
npm init -y
Use code with caution content_copyThe-y
flag tells NPM to accept all default options, creating apackage.json
file for your project. This file manages project dependencies and configurations.
Now you have a basic foundation for building your CLI tool! We’ll dive into the exciting part – creating the actual functionality – in the next steps!
Building Your Core Functionality: Diving into Node.js Scripting
While we can build a functional CLI tool using core Node.js functionalities, there are libraries available that can streamline the process, especially for more complex tools.
Simplifying with CLI Libraries (Commander, Yargs)
For those seeking a smoother development experience, consider using popular CLI libraries like Commander or Yargs. These libraries handle common tasks like argument parsing, user prompts, and help command creation, saving you time and code. Exploring these libraries is recommended for more advanced tools, but for now, we’ll focus on the core functionality using Node.js itself.
Creating the Heart of Your Tool: The Main JavaScript File
- Crafting the Script: Within your project directory, create a JavaScript file to house your tool’s logic. A common naming convention is
index.js
. You can use any text editor or IDE you’re comfortable with. - Accessing User Input: Node.js provides access to command-line arguments passed when running the script. This information is stored in an array called
process.argv
. Let’s explore an example: const arguments = process.argv; console.log(arguments); // This will log all arguments passed to the script - Running this script with arguments like
node index.js copy file1.txt folder2
will log the complete array containing details like the Node.js path, script filename (index.js
), and the provided arguments (copy
,file1.txt
,folder2
). - Understanding Arguments: The first two elements in the
process.argv
array are typically the path to Node.js and the script filename. The actual arguments provided by the user start from the third element onwards. In our example,arguments
would be"copy"
,arguments
would be"file1.txt"
, and so on. - Identifying the Task: By analyzing the arguments array, we can identify the desired task or command. We can use conditional statements (like
if
andelse
) to check the arguments and execute appropriate functionality based on the user’s input.
Building Your Tool’s Core Functionality:
Now that you can access user arguments, let’s showcase how to implement core functionality for a basic tool. Here, we’ll demonstrate two examples: copying a file and renaming a file.
Example 1: Copying a File
const fs = require(‘fs’); // Include the ‘fs’ module for file system access
const sourceFile = arguments[2]; const destinationPath = arguments[3];
if (!sourceFile || !destinationPath) { console.error(‘Please provide both source file and destination path!’); return; // Exit if arguments are missing }
fs.readFile(sourceFile, ‘utf8’, (err, data) => { if (err) { console.error(‘Error reading source file:’, err); return; }
fs.writeFile(destinationPath, data, ‘utf8’, (err) => { if (err) { console.error(‘Error writing to destination:’, err); return; }
console.log('File copied successfully!');
}); });
Explanation:
- We require the
fs
module, which provides functionalities for interacting with the file system. - We extract the source file path and destination path from the arguments array.
- Error handling is implemented to check for missing arguments or file system errors during reading and writing.
- The
fs.readFile
method reads the contents of the source file. - The
fs.writeFile
method writes the copied data to the specified destination path.
Example 2: Renaming a File
const fs = require(‘fs’);
const oldPath = arguments[2]; const newPath = arguments[3];
if (!oldPath || !newPath) { console.error(‘Please provide both the old file path and the new name!’); return; }
fs.rename(oldPath, newPath, (err) => { if (err) { console.error(‘Error renaming file:’, err); return; }
console.log(‘File renamed successfully!’); });
Explanation:
- Similar to the copy example, we require the
fs
module and handle missing arguments. - The
fs.rename
method is used to rename the file at the specifiedoldPath
to the new name provided innewPath
.
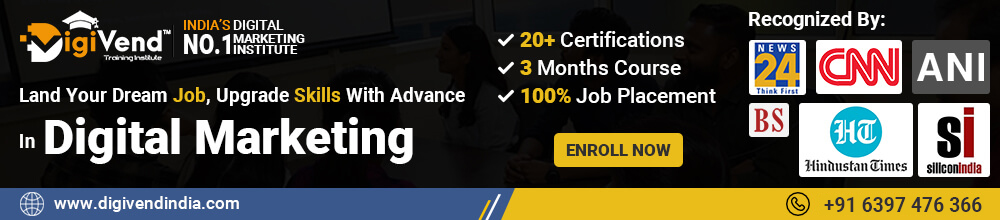