Guide to Setting Up and Configuring Karma for Angular Testing

Testing is an essential part of any software development process. It helps in identifying bugs early, ensuring that the application behaves as expected. Unit testing lets you isolate and test individual components of your application, ensuring they function correctly. In the Angular ecosystem, Karma is one of the most widely used test runners. It works hand-in-hand with Jasmine (for writing tests) and helps developers automate the testing process, saving time and effort.
In this blog, you will go through the process of setting up Karma for Angular testing, including some helpful configuration tips and common issues you might encounter along the way. By the end of this, you’ll be prepared to efficiently write and run tests for your Angular application.
Introduction to Karma for Angular
Karma is a JavaScript test runner built to automate the testing process. It executes your code in multiple browsers and helps you in checking how the code performs in each environment. With Angular, Karma works as part of the Angular CLI, simplifying the setup for developers.
Karma’s integration with continuous integration tools is another reason why it’s a favorite among Angular developers. Once set up, Karma can run your tests automatically every time you make a change to the codebase, giving you feedback in real-time.
Now that you have an idea of what Karma is, let’s dive into how to set it up in your Angular project.
Setting up Karma in an Angular project
To get started, you’ll need to have Node.js and the Angular CLI installed. If you don’t have them installed already, you can grab Node.js from [here](https://nodejs.org/en/download/), and Angular CLI can be installed by running the following command in your terminal:
“`bash
npm install -g @angular/cli
“`
Once you have these installed, you can create a new Angular project using the Angular CLI:
“`bash
ng new my-angular-app
cd my-angular-app
“`
At this point, Karma and Jasmine are already included in the default Angular project setup. So, there’s no need to install Karma manually. You can verify this by checking the `angular.json` file. You should see something like this:
“`json
“test”: {
“builder”: “@angular-devkit/build-angular:karma”,
“options”: {
“main”: “src/test.ts”,
“polyfills”: “src/polyfills.ts”,
“tsConfig”: “tsconfig.spec.json”,
“karmaConfig”: “./karma.conf.js”
}
}
“`
The `karma.conf.js` file contains the configuration for Karma, which we will explore next.
Understanding the karma.conf.js file
This file is where all the Karma configuration lives. Let’s break down the key components:
- BasePath: This sets the base path for resolving files. It’s usually set to an empty string because most projects don’t need a specific base path.
- Frameworks: This is where you define which testing frameworks you want to use. By default, Karma is configured to work with Jasmine for Angular testing.
- Files: This array tells Karma which files to load in the browser for testing. The default configuration includes your test files and source files.
- Browsers: This defines which browsers Karma should run your tests in. The default configuration often includes Chrome, but you can also use Firefox, Safari, or headless browsers like ChromeHeadless.
- Reporters: These are the tools that generate the test results report. By default, Karma uses a “progress” reporter that shows the progress of your tests in the terminal.
Here’s what a basic `karma.conf.js` might look like:
“`js
module.exports = function (config) {
config.set({
basePath: ”,
frameworks: [‘jasmine’, ‘@angular-devkit/build-angular’],
files: [],
preprocessors: {},
browsers: [‘Chrome’],
singleRun: false,
reporters: [‘progress’],
port: 9876,
colors: true,
logLevel: config.LOG_INFO,
autoWatch: true
});
};
“`
You can add more browsers, reporters, and other customizations depending on your project’s needs.
Running tests with Karma
Once Karma is configured, you can run your tests using the following command:
“`bash
ng test
“`
This will trigger Karma to open a Chrome browser (or whichever browser you’ve configured), execute the tests, and provide feedback in the terminal.
If everything is set up correctly, you should see something like:
“`bash
Executed 3 of 3 SUCCESS (0.123 secs / 0.456 secs)
“`
The first number shows the number of tests run, and the second shows the execution time.
Configuration tips for a better Karma experience
Now that we’ve got the basics covered, let’s explore some configuration tips to improve your testing experience with Karma.
1. Use Headless Browsers for CI/CD Pipelines
When running tests on a CI/CD server, you won’t have access to a browser’s graphical interface. This is where headless browsers like ChromeHeadless come in handy. You can modify your `karma.conf.js` file like this:
“`js
browsers: [‘ChromeHeadless’]
“`
2. Improve performance with `singleRun`
By default, Karma watches your files for changes and re-runs tests every time a change is detected. While this is useful for local development, it’s not ideal for CI/CD environments. Set `singleRun` to `true` in your `karma.conf.js` to ensure that Karma runs the tests once and exits:
“`js
singleRun: true
“`
3. Configure code coverage reports
Karma can generate code coverage reports to show how much of your code is covered by tests. You can enable this by adding the `karma-coverage` plugin to your `karma.conf.js`:
“`js
reporters: [‘progress’, ‘coverage’],
“`
Then, add the following preprocessors section:
“`js
preprocessors: {
‘src//.js’: [‘coverage’]
}
“`
This will create a coverage report that you can review to ensure that your tests are covering enough of your codebase.
Common issues with Karma and how to fix them
Like any tool, Karma can occasionally run into issues. Let’s cover some common ones and how to address them.
1. Karma doesn’t launch Chrome
This can happen if Karma can’t find the Chrome executable. You can fix this by specifying the location of Chrome in your `karma.conf.js` file:
“`js
browsers: [‘Chrome’],
customLaunchers: {
Chrome_with_debugging: {
base: ‘Chrome’,
flags: [‘–remote-debugging-port=9222’]
}
}
“`
2. Tests are running slow
If your tests are taking a long time to run, you can switch to a headless browser like ChromeHeadless to speed things up. Another trick is to increase Karma’s concurrency, which allows it to run multiple tests at the same time:
“`js
concurrency: 2
“`
3. Tests aren’t running after modifying a file
If Karma isn’t picking up changes to your files, it might be due to an issue with file-watching. You can force Karma to poll for changes instead of relying on file system events:
“`js
autoWatch: true,
restartOnFileChange: true
“`
Conclusion
Karma is a powerful tool that simplifies automating the testing process for Angular applications. From setting up the basic configuration to addressing common issues, this guide should help you get Karma running smoothly in your project. If you are considering hiring AngularJS developers for app development, they will find that tweaking the configuration to suit your needs can optimize your testing workflow and ensure that your Angular app remains reliable. Remember, if you face challenges, troubleshooting is just part of the learning process. Happy testing!
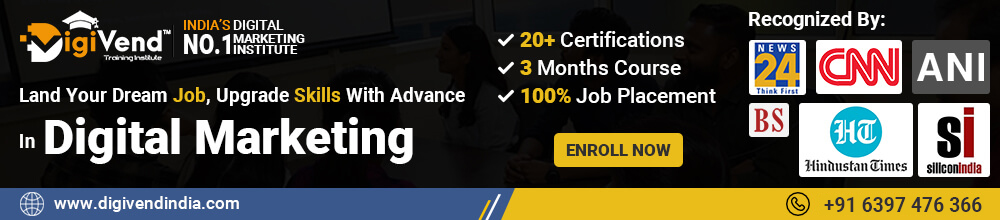